Seller Processing Model (for Platform Use Case)
Process payments with the Seller Processing Model
To process payments under the Platform Use Case, you must adopt the Seller Processing Model, where the Seller Merchant acts as the processing merchant.
Create a Payment
(1) Fund flow logic
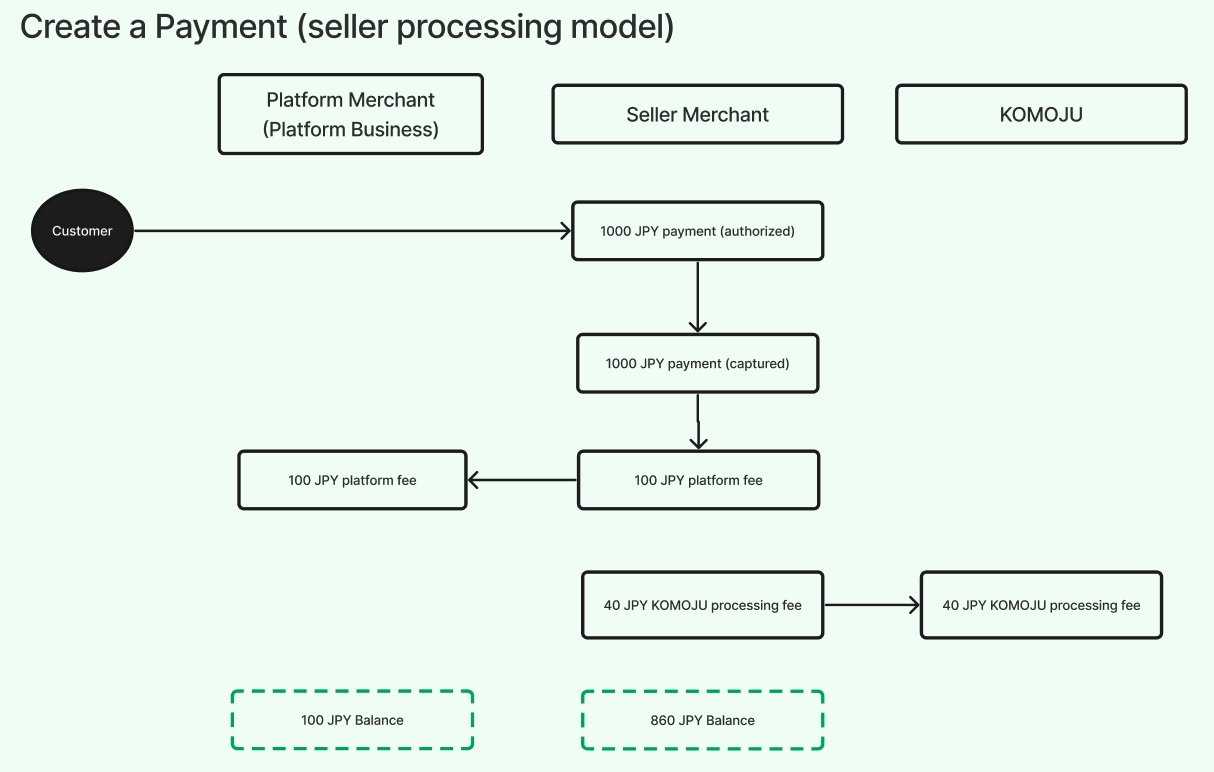
- The Platform Merchant specifies the Platform Fee in each payment, which is charged to the Seller Merchant.
- The Seller Merchant, as the processing merchant, is responsible for paying the Payment Processing Fee to KOMOJU.
(2) Create a payment via API
We support three methods for processing Credit Card payments:
- Direct Payment via API:
- If your platform is PCI-DSS compliant, you can handle card details on your server and send customer payment information directly by using the Payment: Create API. See this guide for more details.
- Tokenization:
If you're not PCI-DSS compliant, use tokenization to avoid handling raw credit card data.- First, request the Token: Create API to tokenize the card details. Refer to this guide for further instructions.
- Once you receive the Token ID, pass it as
payment_details
when creating the payment with the Payment: Create API.
- 3D Secure:
For payments requiring 3D Secure:- Request the SecureToken: Create API to generate a secure token, and redirect customers to
authentication_url
depending on theverification_status
. Refer to this guide for more details. - Then, use the secure token in the Payment: Create API to complete the payment.
- Request the SecureToken: Create API to generate a secure token, and redirect customers to
For non-Credit Card payments, simply use the Payment: Create API with the appropriate customer payment details.
When creating a payment, you must provide the following in the request:
processing_merchant_id
: The ID of the Seller Merchant processing the payment.submerchant_id
andplatform_fee
: The Platform Fee amount to be charged to the Seller Merchant.
"platform_details": {
"processing_merchant_id": "submerchant_one",
"submerchants": [
{
"submerchant_id": "submerchant_one",
"platform_fee": "100",
"amount": "700"
}
]
Request Attribute | Type | Description |
---|---|---|
processing_merchant_id | string | The ID of the Seller Merchant processing the payment. |
submerchant_id | string | The ID of the Seller Merchant. |
platform_fee | integer | The Platform Fee charged to the Seller Merchant. |
amount | integer | The amount deposited to the Seller Merchant. |
Based on the above example, processing_merchant
is submerchant_one
- The
submerchant_one
’s credentials are used to process the payment request - The
submerchant_one
's balance is credited with the payment amount - The
platform_fee
is transferred from thesubmerchant_one
’s balance to the Platform Merchant’s balance - The
submerchant_one
pays the KOMOJU Payment Processing fee
Must specify the payment split logic when capturing paymentsWhen capturing payments, you must provide the payment split information (amount to Seller Merchant and Platform Fee) in the
platform_details
array. This ensures that the payment is split accordingly during capture.
If capture
is set to true
when creating the payment, you must include the payment split in the initial request.
If capture
is false
, you can specify the payment split later when requesting Payment: Capture API.
(3) Create a payment via Hosted Page
KOMOJU's Hosted Page solution provides PCI compliance and 3D Secure by default. It is ideal for platforms that are not PCI-DSS compliant or wish to reduce development effort. Customers are redirected to KOMOJU’s Hosted Page to complete the payment. Learn more in this guide.
To initiate a session for the Hosted Page, use the Session: Create API, and provide the following details:
processing_merchant_id
: The Seller Merchant processing the payment.submerchant_id
andplatform_fee
: The Platform Fee to be charged to the Seller Merchant.
"platform_details": {
"processing_merchant": "submerchant_one",
"submerchants": [
{
"submerchant_id": "submerchant_one",
"platform_fee": "100",
"amount": "700"
}
]
If the capture
mode is set to auto
, you must include the payment split in the initial session creation request.
For manual
capture, the split can be specified later when using the Payment: Capture API for capturing.
Two-step Capture
(1) Authorization
For credit card payments, the Platform Merchant can decide whether to authorize or capture the payment immediately by setting the capture
parameter. If capture: false
is used, the platform split information is not needed during authorization but must be provided during the capture step. However, the processing merchant is required when authorization.
Learn more about two-step capture here.
curl https://komoju.com/api/v1/payments \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
"amount":"1000",
"currency":"JPY",
"fraud_details": {
"customer_ip": "192.0.2.1",
"customer_email": "[email protected]"
},
"payment_details":{
"email":"[email protected]",
"month":"01",
"name":"Taro Yamada",
"number":"4111111111111111",
"type":"credit_card",
"verification_value":"123",
"year":"2025"
},
"capture":"false",
"platform_details":{
"processing_merchant_id":"submerchant_one"
}
}'
(2) Capture
When capturing the payment, you must specify the split information. The combined amount (Seller Merchant’s share and Platform Fee) must not exceed the authorized payment amount.
curl https://komoju.com/api/v1/payments/{PAYMENT_UUID}/capture \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
"amount":"1000",
"platform_details": {
"processing_merchant_id":"submerchant_one",
"submerchants": [
{
"submerchant_id":"submerchant_one",
"amount":"900",
"platform_fee":"100",
}
]
}
}'
(3) Partial Capture
Partial capture is supported for VISA/Mastercard, JCB/AMEX/Diners Club, PayPay, and Merpay. The platform splits must total the amount of the partial capture. KOMOJU supports only a single partial capture per transaction; multiple partial captures are not supported.
If you create a payment via API directly (Payment: Create), partial capture is supported only for VISA/Mastercard and JCB/AMEX/Diners Club.
In contrast, if you create a payment via Hosted Page (Session: Create), all four payment methods support partial capture.
For example, if the original payment was ¥1000, you can partially capture ¥500.
curl https://komoju.com/api/v1/payments/{PAYMENT_UUID}/capture \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
"amount":"500",
"platform_details":{
"processing_merchant_id":"submerchant_one",
"submerchants": [
{
"submerchant_id":"submerchant_one",
"amount":"400",
"platform_fee":"100"
}
]
}
}'
Cancel a Payment
(1) Cancel a Payment via API
You can cancel a payment in a pending
or authorized
state via the Payment: Cancel API.
(2) Cancel a Payment via Dashboard
A payment in the pending
or authorized
state can be canceled on behalf of the Seller Merchant through the Dashboard.
Create a Reverse Refund
(1) Setup
- A Reverse Refund fully refunds the payment to the customer, following the original payment split logic at the time of capture.
- Supported payment methods: VISA/Mastercard, JCB/AMEX/Diners Club, PayPay, Merpay
- The balance for both you and the Seller Merchant must be greater than the respective refund amounts. If either balance is insufficient, the refund will not be processed.
(2) Fund flow logic
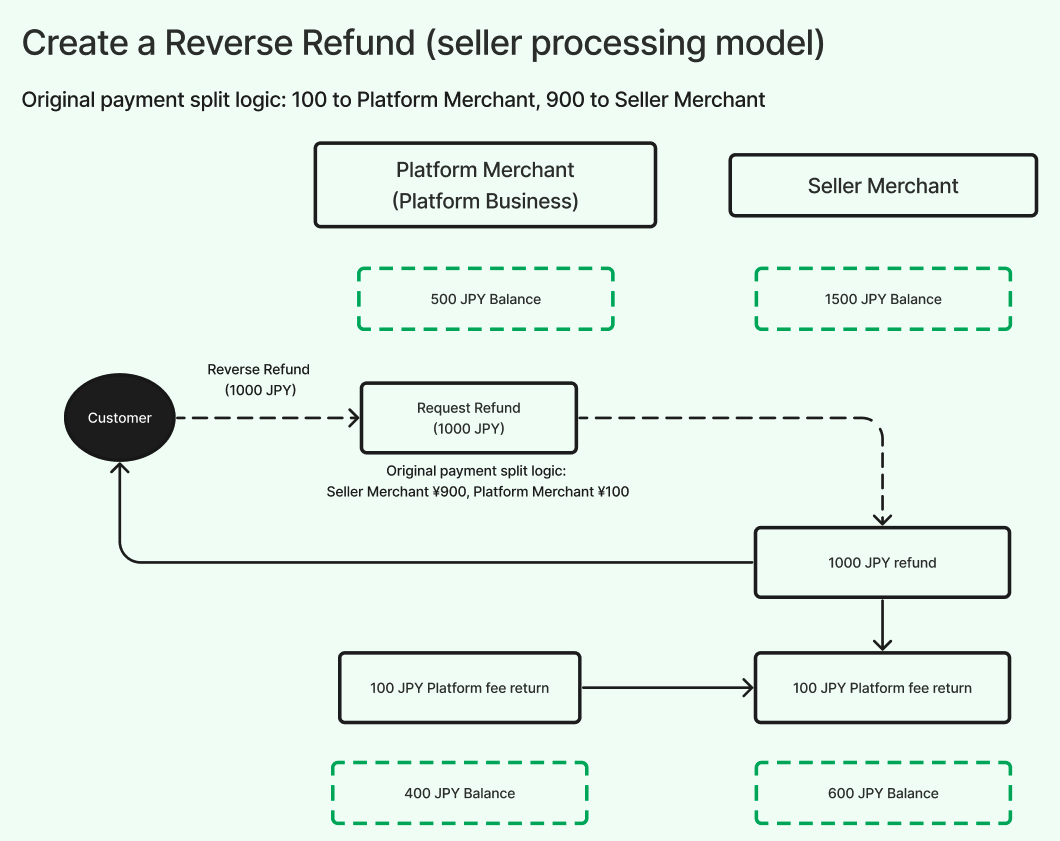
The system debits the funds from the Seller Merchant’s account and refunds the amount to the customer’s account. It then debits the corresponding amount from the Platform Merchant’s account and transfers it to the Seller Merchant’s account.
(3) Create a Reverse Refund via API
You can initiate a Reverse Refund by requesting the Payment: Refund API to initiate a Reverse Refund. The platform details can be omitted, and the system will automatically reverse the splits.
curl https://komoju.com/api/v1/payments/{PAYMENT_UUID}/refund \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
}'
(4) Create a Reverse Refund via Dashboard
A Reverse Refund can be initiated on behalf of the Seller Merchant by using the Dashboard.
Create a Reverse Refund Request
(1) Setup
- A Reverse Refund Request is for payment methods that do not support automatic refunds. This will fully refund the payment to the customer based on the payment split logic at the time of capture.
- Supported payment methods: Konbini, Bank Transfer, and Pay-Easy
- The balance for both you and the Seller Merchant must be greater than the respective refund amounts. If either balance is insufficient, the refund will not be processed.
- You must collect the customer's bank account details and provide them to KOMOJU for processing
- KOMOJU charges ¥300 per successful refund from the Seller Merchant for each Reverse Refund Request. (reference)
- If a Customer Fee was charged in the original payment, you can choose whether to refund it using the
include_payment_method_fee
parameter.
(2) Fund flow logic
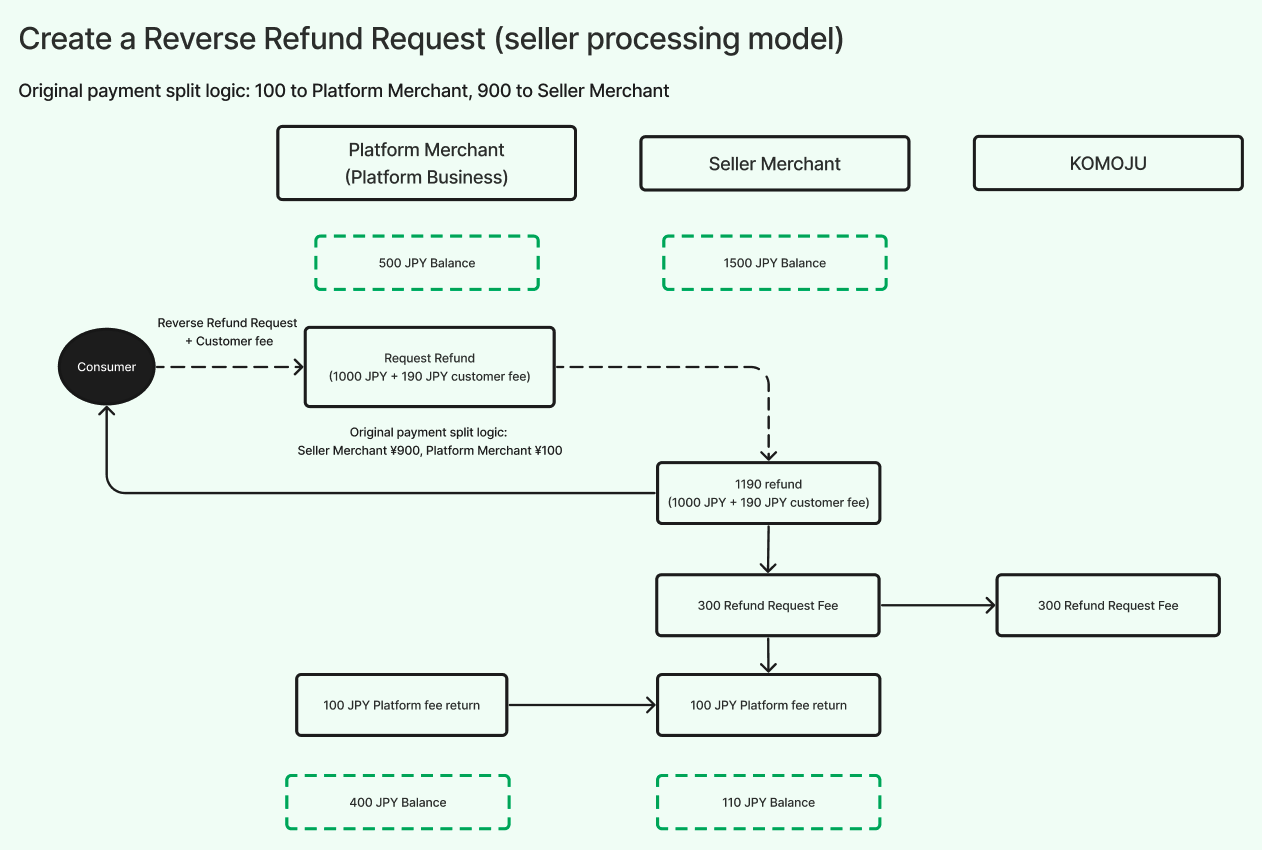
The system debits the funds from the Seller Merchant’s account and refunds the amount to the customer’s account. It then debits the corresponding amount from the Platform Merchant’s account and transfers it to the Seller Merchant’s account.
(3) Create a Reverse Refund Request via API
You can request the Payment: Refund Request API to initiate a Reverse Refund Request along with the customer's bank account information. The platform details can be omitted, and the system will automatically reverse the splits.
curl https://komoju.com/api/v1/payments/{PAYMENT_UUID}/refund_request \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
"include_payment_method_fee":"true",
"customer_name":"カタカナ",
"bank_name":"青森銀行",
"bank_code":"0117",
"branch_name":"東京支店",
"branch_number":"921",
"account_type":"ordinary",
"account_number":"1234567",
}'
(4) Create a Reverse Refund Request via Dashboard
A Reverse Refund Request can be executed on behalf of the Seller Merchant through the Dashboard.
Create a Non-Reverse Refund
(1) Setup
A Non-Reverse Refund should be used when
- A full refund does not follow the original payment split logic
- A partial refund is required
Notes:
- The refund amount can not exceed the original payment amount.
- Supported payment methods: VISA/Mastercard, JCB/AMEX/Diners Club, PayPay, Merpay
- Both you and the Seller Merchant must have sufficient balances to cover the refund amounts in charge.
(2) Fund flow logic
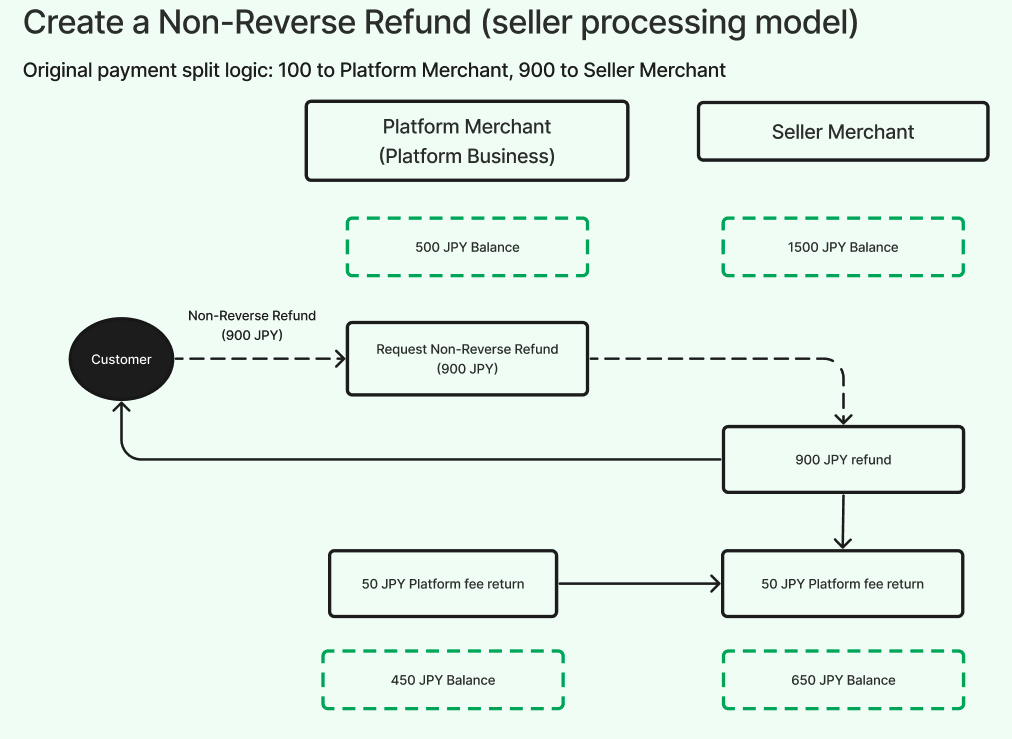
You specify the refund amounts for both you and the Seller Merchant. The system debits the Seller Merchant's account and refunds the customer. It then debits the corresponding amount from the Platform Merchant and transfers it to the Seller Merchant.
In this example,
- A ¥1,000 payment was split into two pieces when capturing the payment: ¥900 to Seller Merchant, and ¥100 to Platform Merchant originally.
- Later, Platform Merchant requests a Non-Reverse Refund and specifies a total refund ¥900 to the customer, which is composed of ¥850 from the Seller Merchant and ¥50 from the Platform Merchant.
(3) Create a Non-Reverse Refund via API
A Non-Reverse Refund can be initiated only via the Payment: Refund API to avoid manual errors.
curl https://komoju.com/api/v1/payments/{PAYMENT_UUID}/refund \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
"amount":"900",
"platform_details":{
"processing_merchant_id":"submerchant_one",
"submerchants": [
{
"submerchant_id":"submerchant_one",
"amount':"850",
"platform_fee":"50",
}
]
}
}'
Create a Non-Reverse Refund Request
(1) Setup
A Non-Reverse Refund Request should be initiated in the following cases:
- When issuing a full refund that does not follow the original payment split logic.
- When issuing a partial refund.
Notes:
- The refund amount can not exceed the original payment amount.
- Supported payment methods: Konbini, Bank Transfer, and Pay-Easy
- Both your balance and the Seller Merchant's balance must cover the respective portions of the refund. If either balance is insufficient, the refund will not be processed.
- You must collect the customer’s bank account details and provide them to KOMOJU, allowing the customer to receive the refund.
- KOMOJU charges a ¥300 fee for each successful Non-Reverse Refund Request, deducted from the Seller Merchant. (reference)
- If the Seller Merchant charged a Customer Fee in the original transaction, you can specify whether to refund it using the
include_payment_method_fee
parameter.
(2) Fund flow logic
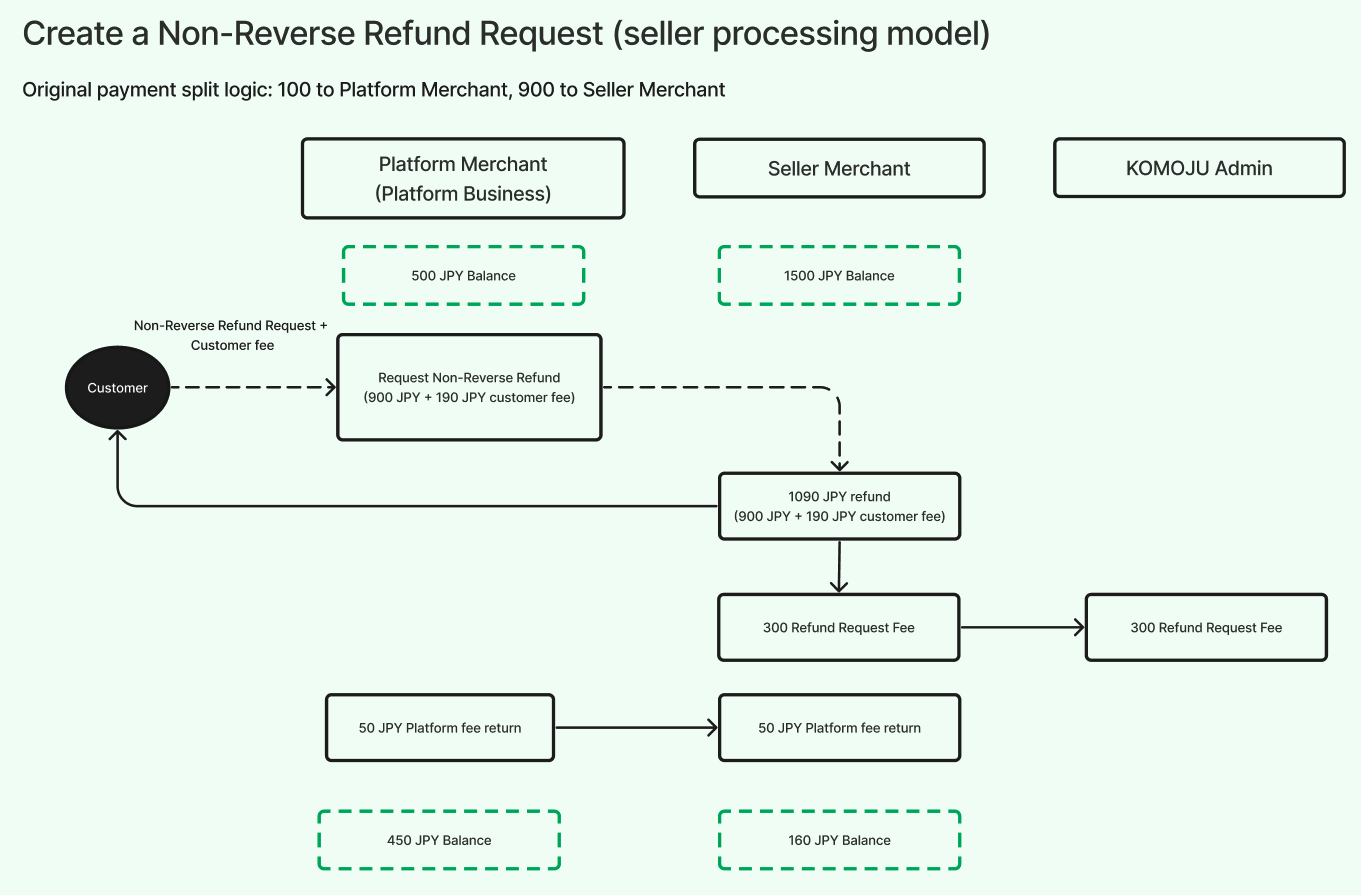
When issuing a Non-Reverse Refund Request via API, you must specify the amounts to be refunded by both the Seller Merchant and you. The system debits the refund amount from the Seller Merchant’s account and refunds it to the customer’s bank account. Then, the system debits the corresponding amount from the Platform Merchant’s account and transfers it to the Seller Merchant’s account.
For example,
- A ¥1,000 payment was split into two parts: ¥900 to the Seller Merchant and ¥100 to the Platform Merchant.
- The Platform Merchant initiates a Non-Reverse Refund Request for ¥900, consisting of ¥850 from the Seller Merchant and ¥50 from the Platform Merchant.
- KOMOJU deducts a ¥300 refund fee from the Seller Merchant.
(3) Create a Non-Reverse Refund Request via API
Use the Payment: Refund Request API to initiate a Non-Reverse Refund Request. You must also provide the customer's bank account information. This type of refund cannot be processed via the Dashboard to avoid manual errors.
curl https://komoju.com/api/v1/payments/{PAYMENT_UUID}/refund_request \
-u degica-mart-test: \
-X POST \
-H "Content-Type: application/json" \
-d '{
"amount":"900",
"include_payment_method_fee":"true",
"customer_name":"カタカナ",
"bank_name":"青森銀行",
"bank_code":"0117",
"branch_name":"東京支店",
"branch_number":"921",
"account_type":"ordinary",
"account_number":"1234567",
"platform_details":{
"processing_merchant_id":"submerchant_one",
"submerchants": [
{
"submerchant_id":"submerchant_one",
"amount':"850",
"platform_fee":"50",
}
]
}
}'
Chargeback
(1) Setup
If the Seller Merchant loses a dispute, as determined by the card issuer, a chargeback will be executed. The entire payment will be returned to the customer, and the corresponding amount will be debited from the Seller Merchant's balance. The chargeback will proceed regardless of whether the Seller Merchant’s balance is sufficient.
(2) Fund flow logic
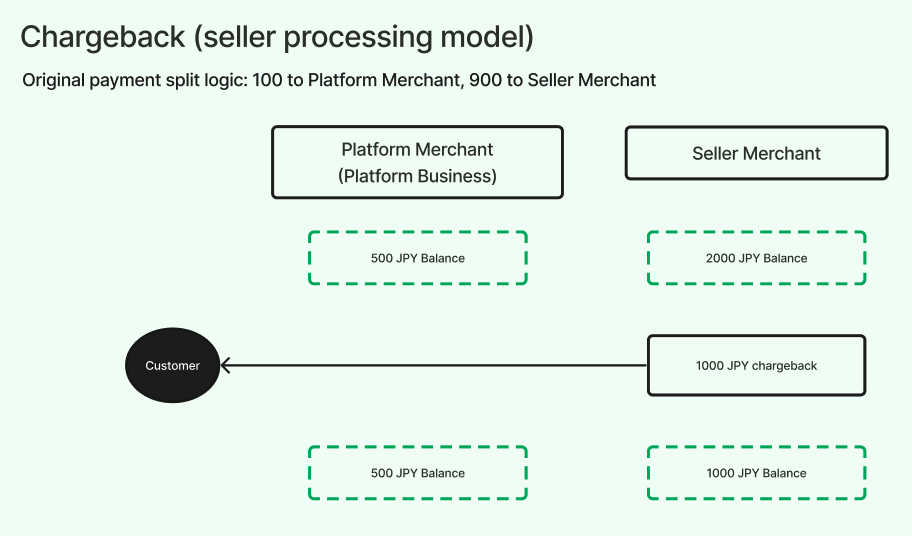
Since the Seller Merchant is the processing merchant, it is responsible for the entire chargeback amount.
Summary of transaction record types
The following table summarizes the types of records generated based on various actions:
Record type | Description | Deposit/Debit |
---|---|---|
Payment | The payment captured under Seller Merchant's account. | (1) Seller Merchant: Deposit |
Platform Fee | The fee that Platform Merchant charges the Seller Merchant for each captured payment. | (1) Platform Merchant: Deposit (2) Seller Merchant: Debit |
Processing Fee | The fee KOMOJU charges the Seller Merchant for each captured payment. The rate varies by payment method. | (1) Seller Merchant: Debit |
Processing Fee Tax | The tax on the Processing Fee. In Japan, this is 10% of the Processing Fee amount. | (1) Seller Merchant: Debit |
Refund | The amount the Seller Merchant refunds to a customer. | (1) Seller Merchant: Debit |
Platform Fee Return | The amount of Platform Fee the Platform Merchant returns to the Seller Merchant, representing shared responsibility in a refund. | (1) Platform Merchant: Debit (2) Seller Merchant: Deposit |
Refunded Customer Fee | The Customer Fee refunded by the Seller Merchant to the customer. | (1) Seller Merchant: Debit |
Refunded Customer Fee Tax | The tax on the Customer Fee refunded by the Seller Merchant. In Japan, this is 10% of the fee amount. | (1) Seller Merchant: Debit |
Refund Fee | The fee KOMOJU charges the Seller Merchant for a successful Reverse Refund Request or Non-Reverse Refund Request. | (1) Seller Merchant: Debit |
Refund Fee Tax | The tax on the Refund Fee. In Japan, this is 10% of the Refund Fee amount. | (1) Seller Merchant: Debit |
Chargeback | The amount the Seller Merchant refunds to a customer after losing a dispute, as determined by the card issuer. | (1) Seller Merchant: Debit |
Updated about 1 month ago