React Native
KOMOJU offers a fully localized React Native SDK for seamless payment integration
The KOMOJU React Native SDK securely processes payments within your mobile application.
If you are using the SDK UI, it communicates directly with our backend when handling credit card information minimizing your PCI-DSS burden.
Additionally, our React Native SDK supports a variety of payment methods, including Konbini and PayPay in Japan, enabling you to cater to your customers' preferred payment options.
The source code is freely available at GitHub and includes a starter application for testing.
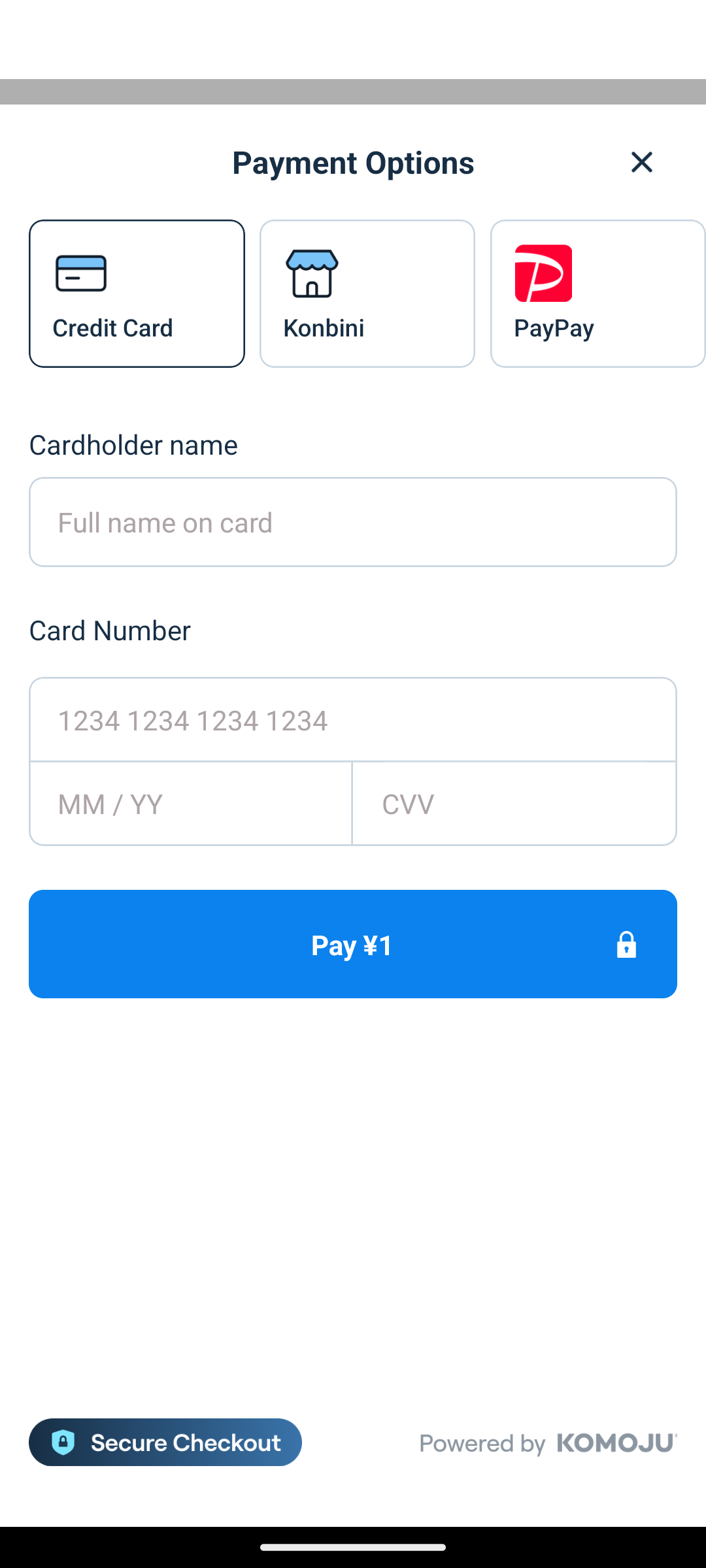
Getting Started
Install the @komoju/komoju-react-native package into your React Native application
yarn add @komoju/komoju-react-native
or
npm install @komoju/komoju-react-native
Payment Flow
The payment flow involves four main steps: creating a session, fetching the session and initializing the SDK, handling the payment completion callback, and receiving payment.captured
Webhook.
The diagram below illustrates the overall process,
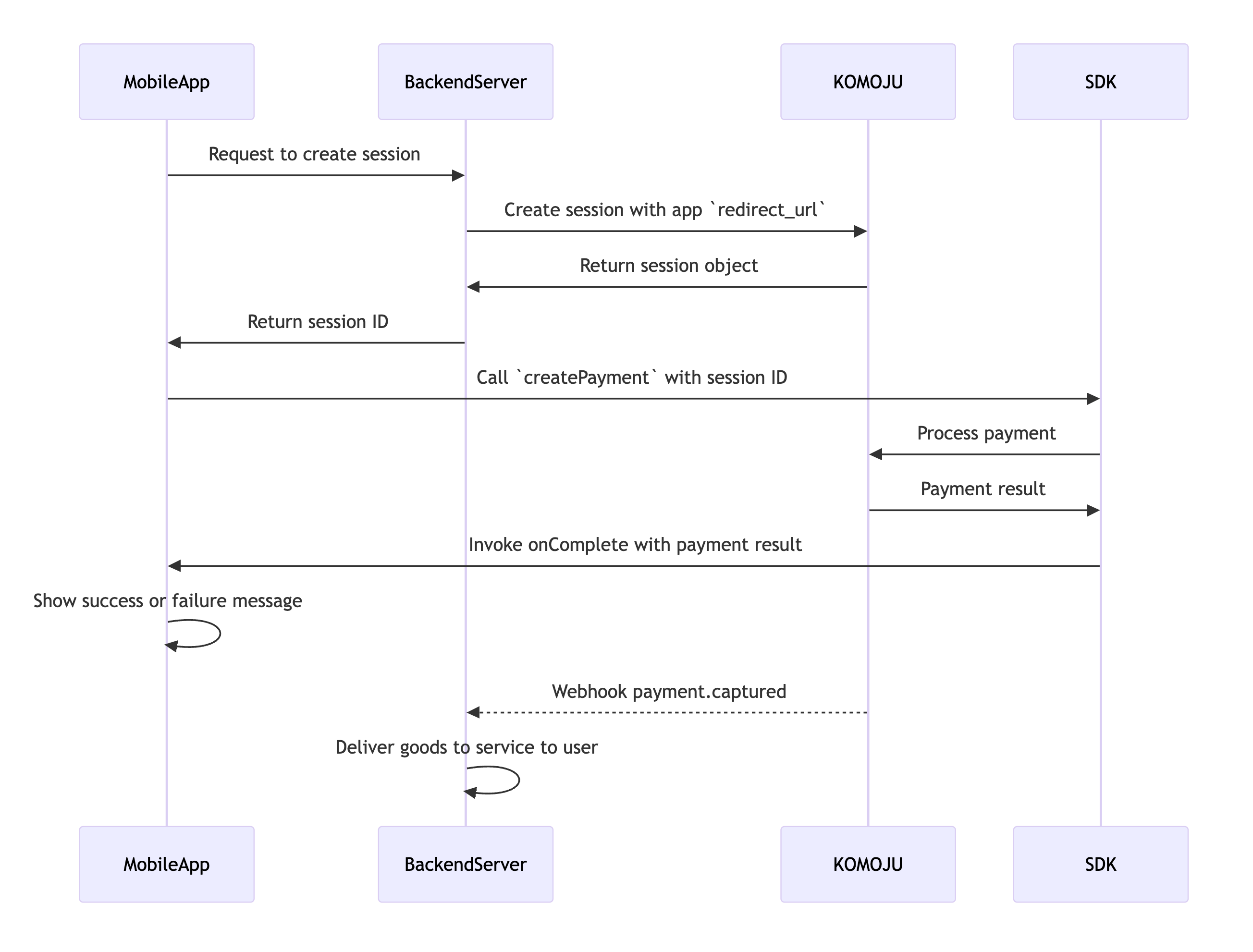
1. Creating a session
Setup a secure endpoint and create a Session Object on your backend server (see example).
When creating the session you will need to set the redirect_url
to point to your app URL scheme e.g. myapp://
Configure the custom URL scheme in your AndroidManifest.xml and Info.plist files. Note: If you’re using Expo, set your scheme in the app.json file.
2. Fetch the session and create a payment
Call your session endpoint and create a payment by passing sessionId
to createPayment
(see script example in SDK Initialization)
3. Setup your completion callback
When initializing the SDK you will need to setup onComplete
handler. When the customer returns back the handler will be called with the updated session object.
const onComplete = (session: SessionShowResponseType) => {
if (session.status == "completed") {
console.log(`Thanks! Your payment status is ${session.payment.status}`)
} else {
console.error("Sorry! You've had a problem paying.")
}
}
4. Handle payment.captured
webhook
payment.captured
webhookOn your backend server we advise setting up a Webhook to listen to the final payment.captured
status which indicates the payment is paid. There may be a timeout or exception during the payment process so this event ensures the users payment is finalized. Note, this event is sent asynchronously.
SDK Initialization
In order to use the SDK you will need to initialize KomojuSDK.KomojuProvider
with the following properties,
Property | Type | Description |
---|---|---|
publishableKey | string | The publishable key of your merchant |
paymentMethods | Array<PaymentTypes> | Explicitly set the payment method(s) for purchase. If not, will show all available payment methods on the merchant (optional) |
language | string (LanguageTypes) | Explicitly set the language, if not set language will be picked from your session Id (optional) |
You will then need to wrap the component around your payment screen as shown below,
// App.ts
import {
KomojuSDK,
PaymentTypes,
LanguageTypes,
} from "@komoju/komoju-react-native";
function App() {
return (
<KomojuSDK.KomojuProvider
publishableKey={publishableKey}
// optional properties
payment_methods={[PaymentTypes.KONBINI]}
language={LanguageTypes.JAPANESE}
>
<PaymentScreen /> // your payment screen component
</KomojuSDK.KomojuProvider>
);
}
// PaymentScreen.ts
import { KomojuSDK } from "@komoju/komoju-react-native";
export default function PaymentScreen() {
const { createPayment } = KomojuSDK.useKomoju();
const onComplete = (session: SessionShowResponseType) => {
if (session.status === 'completed') {
console.log('Thanks! Your payment is ' + session.payment.status)
} else {
console.log("Sorry! You must have had a problem paying.")
}
}
const onDismiss = (session: SessionShowResponseType) => {
console.log("User has closed the payment dialog");
};
const checkout = async () => {
try {
createPayment({
sessionId, // retrieved from your server
onComplete, // invoked with the updated session after user completes or cancels session
onDismiss, // invoked when the dialog is closed by the user
});
} catch (error) {
console.error("Payment initiation failed:", error);
}
};
return (
<View>
<Button title="Checkout" onPress={checkout} />
</View>
);
}
Updated 2 months ago