Android
KOMOJU offers a fully localized Android SDK that enables smooth payment integration within your android mobile app.
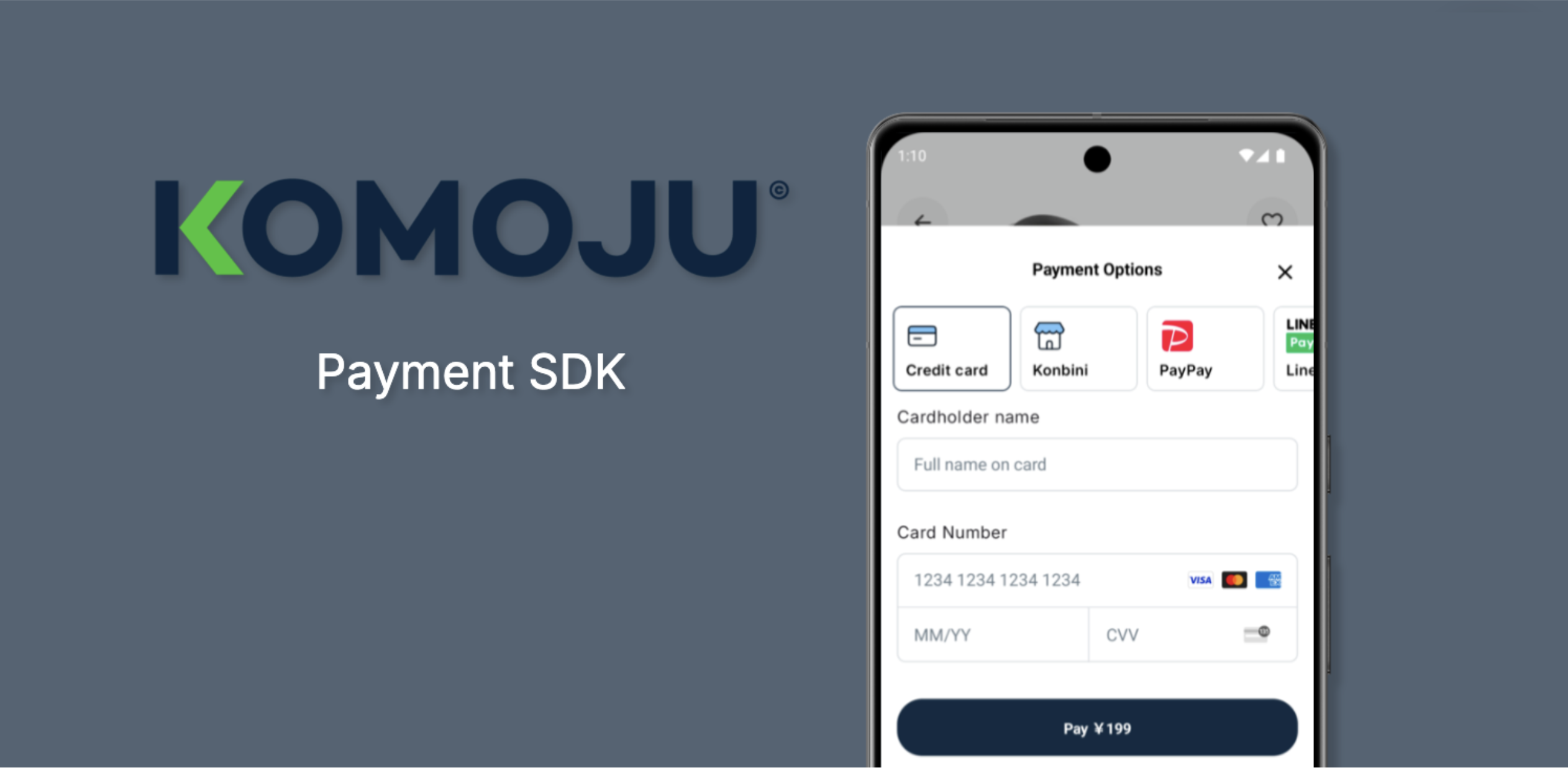
Komoju payment SDK
What it is?
The KOMOJU Android SDK processes payments securely by having its UI communicate directly with our backend to handle sensitive credit card data, reducing your PCI-DSS compliance burden.
What payment method are supported?
Moreover, the Android SDK supports multiple payment methods, including Konbini and PayPay in Japan, enabling you to cater to your customers' preferred payment options.
Show me the code
The source code is freely available on GitHub and includes a sample application for easy testing and setup.
Payment Flow
The payment flow consists of four key steps: creating a session, retrieving the session and initializing the SDK, managing the payment completion callback, and receiving the payment.captured webhook.
The diagram below outlines the entire process.
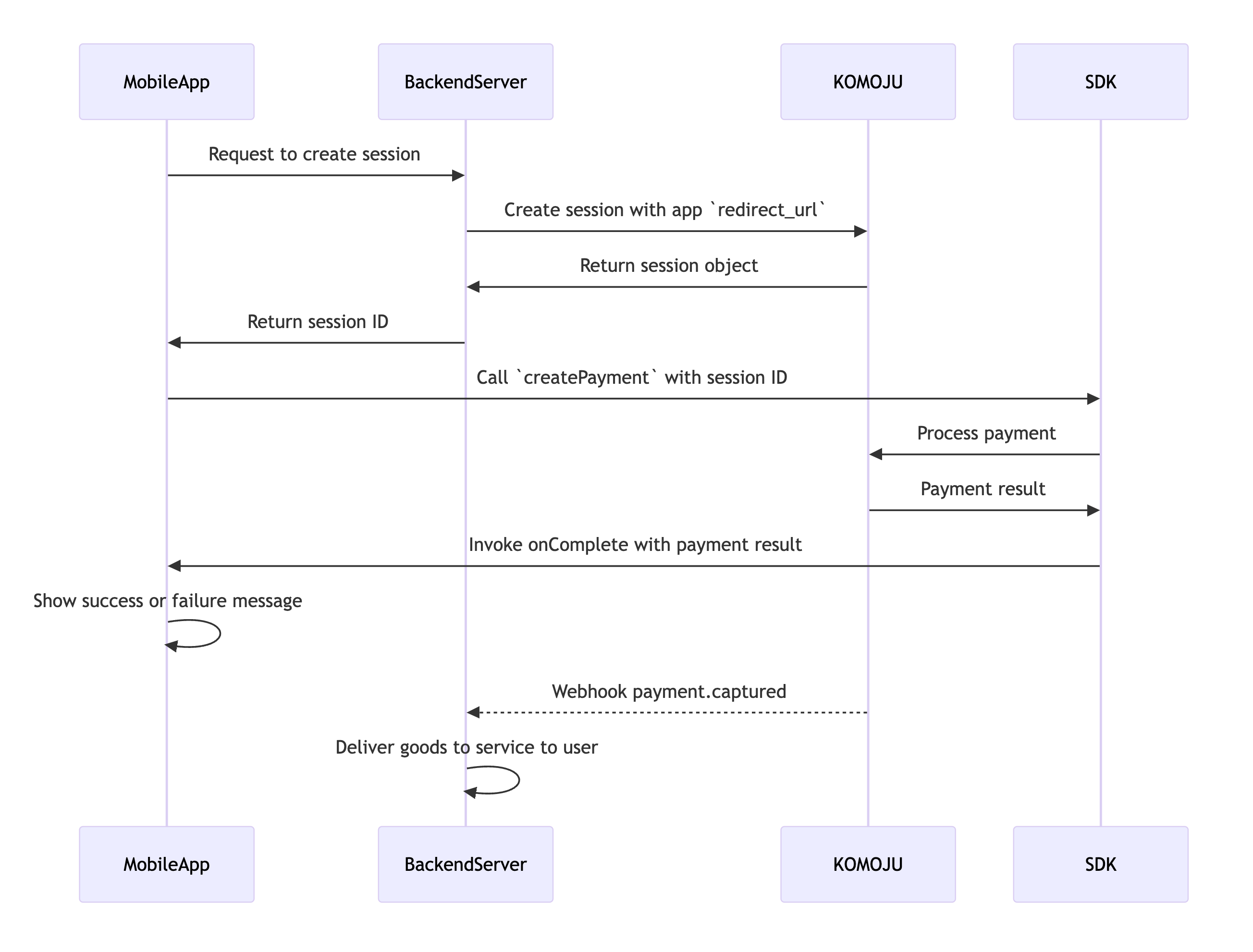
Demo
There is a prebuilt demo named Soundbud! available to check the SDK in action, get the latest build from the Github release page from the Assets section.
Getting Started
Requirements
- Android API 24+
- Gradle 8.X.X+
- The Android SDK utilize the latest version of jetpack compose.
Add the below dependency to your build script as per provided SDK version.
implementation("com.komoju.mobile.sdk:android:<latest-version-here>")
implementation 'com.komoju.mobile.sdk:android:<latest-version-here>'
[versions]
komojuAndroid = "<latest-version-here>"
[libraries]
komoju-mobile-sdk-android = { group = "com.komoju.mobile.sdk", name="android", version.ref = "komojuAndroid" }
// build.gradle.kts
implementation(libs.komoju.mobile.sdk.android)
Creating a session
Set up a secure endpoint and generate a Session Object on your backend server (refer to the example).
While creating the session, ensure that the redirect_url points to your app's URL scheme, e.g., myapp://. and add the schema to your strings.xml or the build script like below.
// Either in build script
resValue("string", "komoju_consumer_app_scheme", "myapp")
// Or in your strings.xml
<string name="komoju_consumer_app_scheme">myapp</string>
Prepare SDK Launcher
val komojuPaymentLauncher = rememberLauncherForActivityResult(KomojuSDK.KomojuPaymentResultContract) { paymentResult ->
if (paymentResult.isSuccessFul) {
// Handle success
} else {
// Handle failure
}
}
private val komojuPaymentLauncher = registerForActivityResult(KomojuSDK.KomojuPaymentResultContract) { paymentResult ->
if (paymentResult.isSuccessFul) {
// Handle success
} else {
// Handle failure
}
}
private final ActivityResultContract<KomojuSDK.Configuration, KomojuSDK.PaymentResult> activityResultContract = KomojuSDK.getKomojuPaymentResultContract();
private final ActivityResultLauncher<KomojuSDK.Configuration> komojuPaymentLauncher = registerForActivityResult(activityResultContract, paymentResult -> {
if (paymentResult.isSuccessFul()) {
// Handle success
} else {
// Handle failure
}
});
Create KomojuSDK Configuration
val komojuSDKConfigurationBuilder = KomojuSDK.Configuration.Builder(
// The Publishable Key for the merchant
publishableKey = publishableKey,
// Created session Id from Your server
sessionId = sessionId,
)
// Optional configuration
// Set SDK Language, Default is System language
komojuSDKConfigurationBuilder.setLanguage(language)
// Set SDK Currency, Default is Japanese
komojuSDKConfigurationBuilder.setCurrency(currency)
// Set SDK Theme, Default is [DefaultConfigurableTheme]
komojuSDKConfigurationBuilder.setConfigurableTheme(theme)
// Set if SDK should process credit card payment inlined, Default is false
// This one is experimental and require OptIn `ExperimentalKomojuPaymentApi`
komojuSDKConfigurationBuilder.setInlinedProcessing(boolean)
val komojuPaymentConfiguration = komojuSDKConfigurationBuilder.build()
KomojuSDK.Configuration.Builder komojuSDKConfigurationBuilder = new KomojuSDK.Configuration.Builder(
// The Publishable Key for the merchant
"publishableKey",
// Created session Id from Your server
"sessionId");
// Optional configuration
// Set SDK Language, Default is System language
komojuSDKConfigurationBuilder.setLanguage(language);
// Set SDK Currency, Default is Japanese
komojuSDKConfigurationBuilder.setCurrency(currency);
// Set SDK Theme, Check Theme
komojuSDKConfigurationBuilder.setConfigurableTheme(theme);
// Set if SDK should process credit card payment inlined, Default is false
// This one is experimental and require OptIn `ExperimentalKomojuPaymentApi`
komojuSDKConfigurationBuilder.setInlinedProcessing(boolean);
KomojuSDK.Configuration komojuPaymentConfiguration = komojuSDKConfigurationBuilder.build();
Launch Payment SDK
val komojuPaymentLauncher = // Created from Step 1
val komojuPaymentConfiguration = // Created from Step 2
if (komojuPaymentConfiguration.canProcessPayment()) {
komojuPaymentLauncher.launch(komojuPaymentConfiguration)
}
private final ActivityResultLauncher<KomojuSDK.Configuration> komojuPaymentLauncher = // Created from Step 1
private final KomojuSDK.Configuration komojuPaymentConfiguration = // Created from Step 2
if (KomojuSDKKt.canProcessPayment(komojuPaymentConfiguration)) {
komojuPaymentLauncher.launch(komojuPaymentConfiguration);
}
The result will be captured in the komojuPaymentLauncher
callback which we created on step 1
SDK Theme
A few ui components of the SDK are configurable, Please refer to the below table
param | sets | returns |
---|---|---|
primaryColor | Color value for the primary components like pay button. | An integer representing a color value |
primaryContentColor | Color value for the content (e.g., text or icon) inside the primary component. | An integer representing a color value |
primaryButtonCornerRadiusInDP | Corner radius for the primary button in density-independent pixels (DP). | An integer representing the corner radius in DP |
loaderColor | Color value for the loader/spinner. | An integer representing a color value |
Default theme can be seen below.
data class DefaultConfigurableTheme(
override val primaryColor: Long = 0xFF297FE7, // Default blue color for primary button.
override val primaryContentColor: Long = 0xFFFFFFFF, // Default white color for primary button content.
override val primaryShapeCornerRadiusInDp: Int = 8, // Default corner radius of 8 DP for primary button.
override val loaderColor: Long = 0xFF3CC239, // Default green color for loader.
) : ConfigurableTheme
To Override the default theme, Please copy the default theme object and pass it to the KomojuSDK.Configuration Builder, check the example below.
val myAppThemeForKomojuSDK = ConfigurableTheme.default.copy(
primaryColor = Color.BLUE,
primaryContentColor = Color.WHITE,
primaryButtonCornerRadiusInDP = 24,
loaderColor = Color.GREEN,
)
// Set SDK Theme, Check Theme
komojuSDKConfigurationBuilder.setConfigurableTheme(theme)
Issues
If you face any issue or have a feedback, Feel pre to report it on github.
Updated 4 months ago